Software Design and Implementation
T2: Exploring Turtles in Python
Objectives:
- Learn a bit about open source software
- Learn some important object-oriented programming (OOP) terminology in computer science
- Begin to learn to use turtle graphics in Python
Team assignment T2 should be explored in class with a pair partner from your team. Use your pair partner to talk together if anything is confusing. Then when we get to the point of submitting, we will take on roles.
Graphics in Python via Turtles
One of the wonderful things about Python is that it is open-source, so there are many authors who have written many modules (aka libraries) that provide powerful features. Many of these modules have become part of the standard Python install. Today's exploration is dedicated to creating graphics via a module called the turtle module.Before we can get started, we need to digress.
Open-Source Software
Open source software is software where the original source code is made freely available and which may be redistributed and modified. Python is open source. Much great software is open-source: Linux, Firefox, Python, Wordpress, etc.When proprietary software breaks, you get security breaches, patches, and updates, but you cannot fit it yourself or even look at the code. When open-source software breaks, you still might get patches and updates from others, but you can look at the code, and you might try to fit it yourself!
So why I am telling you this? Spyder does not seem to play nice with the screen class of the turtle module. A program which uses the screen object will run fine the first time, but then when you try to close the screen, Spyder leaves the screen in an incorrect state. So, we have two choices, switch IDEs or patch the problem. At this point, I suggest we patch it.
Here are several programs which are correct (basically from our textbook) and which should run (download by right-clicking):
Download any of these and run. It should run and display a graphics
screen (window) which might end up behind the IDE.
If you run turtle-first.py
you should see something like:
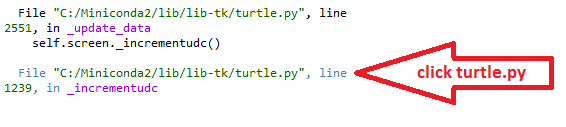
I found that simply recompiling python.py took care of this problem. You could also get drastic and comment out the actual problem (mine was on line 1239) as follows.
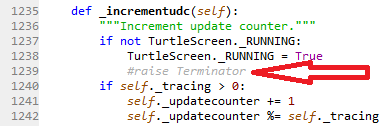
Either way, if you have this problem, you will want to run python.py, so you will need to open it by clicking on the link in the error message to python.py.
We will do this in class. When running python,.py, you should see some nice images such as:
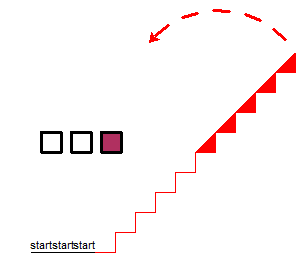
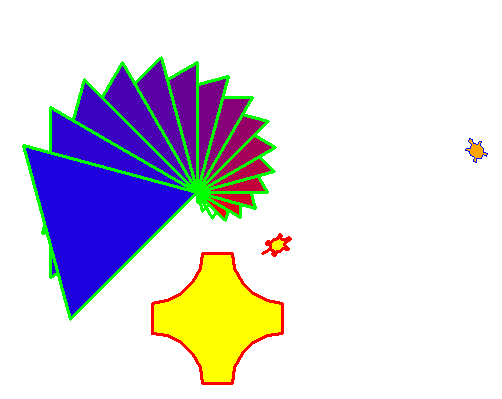
Once you have run python.py, go back and re-run turtle-first.py. Hopefully, the problem is now solved!
Object-oriented Terminology
Python supports an important kind of program design called object-oriented programming. I want to take this opportunity to introduce some important terminology: classes, objects, states, and methods. According to people who track these things, Java, C, C++, C#, and Python are currently the top five programming languages. Four of these languages support are object-oriented programming design techniques. In an object-oriented language, we leverage the object metaphor to organize our programs.At this point, we have already used a class, namely the random class. We are about to use a couple more: the turtle class and the screen class. A class can be thought of as a template or a blueprint that describes objects of its type and the states as well as the behaviors that an object of its type supports. Hence, an object is simply a particular instance of a class. By using the turtle as a metaphor, we can better understand a number of importance computer science concepts.
State is a term in computer science which means a specific description of an object at a given point in time. You can imagine a turtle as an object whose tails is a pen. The state of the turtle would include such things as where it is located on the screen, how fast it is moving, which way it is traveling, what color the turtle is, etc. When the turtle moves around on the screen drawing, the state changes. Similarly, the window object has a background color which is part of its state.
An object (such as a turtle) has various things it can do (called methods) and it can also have inherent properties (called attributes). Quite a number of methods exist that allow us to modify the states of the turtle and window objects. A special method called a constructor method is used before any other in order to create a new particular instance of an object. The following commands use the constructors to make instances of the specific object:
wn = turtle.Screen()
tess = turtle.Turtle()
Once it is created, the turtle object supports behaviors such as moving forward, turning left, etc which are changed or initiated with by calling appropriate methods. One can also use methods to change some of the attributes. For example, each turtle has a color attribute. The method invocation
tess.color("red")
will
make the instance of the specific turtle object named tess turn red,
so the line that it draws will be red too.Okay, that is probably enough with terminology for today. I expect you want to have some fun with turtles.
The Requirements and Deliverables
For the remainder of this teamwork, please use the following roles:- Driver/Facilitator: Reads the questions aloud, keeps track of time, and makes sure both partners contribute appropriately.
- Navigator/Recorder: Fully contributes in discussions then records all questions and answers.
- If there is a third partner, they should serve as a Quality Control Officer: Considers how the answers could be deeper, and how the team could work and learn more effectively.
- Teamwork T2 Exploring OOP and
Turtles
- Driver/Facilitator: Matt Jadud
- Navigator/Recorder:
Jan Pearce
- Followed by answers to all of the following questions:
- Run all of the programs following programs: ere are several
programs which are correct (basically from our textbook) and which
should run (download by right-clicking):
List all of the different methods of the screen objects used in the above programs, and briefly describe how each affects the state of the screen. - List all of the methods of
the turtle used in these programs, and briefly describe how each
affects the state of the turtle.
- Next the Driver/Facilitator should rename one of the above
programs yourusername(s)-T2.py and do the following:
- Put in the following
header comment with the name of the assignment, and the names
and roles of all team members in a header at the top of the
program. For example:
'''Teamwork T2 Exploring OOP and Turtles
Driver/Facilitator: Matt Jadud
Navigator/Recorder: Jan Pearce'''
- Make your program draw at least two letters which represent your teamname on the screen. My team is the Jadud-Pearce team with initials JP.
- After you get at lest two letters which represent initials
drawn, make it as fancy as you have time for. Here is mine:
- Be sure to add comments to each section (or chunk) of your code.
- Put in the following
header comment with the name of the assignment, and the names
and roles of all team members in a header at the top of the
program. For example:
- Describe your team's reaction to the teamwork. Did you achieve the learning objectives listed at the top of the page? How might I consider ways of making it even better in the future?