Software Design and Implementation
T3: Boustrophedon Turtles
Paired assignment T3 should be completed with a partner. Please change driver/navigator pairs. If you were the driver last time, please navigate this time. If you were the navigator last time, please drive this time.
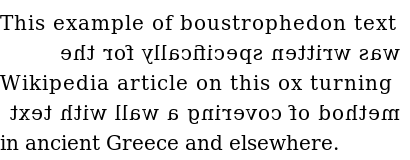
In this assignment, your will design two different algorithms which
use the Python Turtle library to draw a rectangle and fill that rectangle using a boustrophedon pattern. These algorithms must substantially differ. i.e. just changing the colors or the text would not be sufficient.
Boustrophedon pattern using a Python Turtle
The Python Turtle screen has default x-coordinate horizontal width of 683 pixels and default y-coordinate vertical height of 576 pixels. The default setting for (0, 0) is in the center of this screen.The following video uses a pensize size of 20. It first draws a purple square with sides of 500 pixels around the center. It then fills using a green horizontal boustrophedon pattern, and finishes using black text.
Here is an implementation of a second algorithm:
Useful Stuff on Functions and Turtle Methods
Here are some examples of code which is organized into functions:
The following methods were particularly useful for a turtle named myturtle
:
myturtle.left(90)
myturtle.right(90)
myturtle.forward(distance)
myturtle.penup()
myturtle.setpos(xcoord,ycoord) # use to move turtle to a particular (x, y) screen coordinate
myturtle.pendown()
myturtle.color(newcolor)
The instructions
This Paired Assignment has several steps:- Create a new file called yourusernames-T3.docx. This will serve as a report for this team assignment. Be sure to put both names on the top.
- In the Word document, design two different algorithms which could be used to draw a large square and fill it using non-overlapping, just touching boustrophedon patterns as shown in the videos.
- You MUST use functions for encapsulating each "mental chunk".
- Include a
main()
function. - Make effective use of functions and to use docstrings to help clearly explain what each function is designed to do (hopefully, what the functions do will match what you wrote they would do).
- The highest level of your program (i.e., no indenting) should only
contain the following:
- the header
- any import statements
- function definitions
- a call to the main() function
- All of your own function definitions should come before the
def main():
function definition AND the call to themain()
function. In other words, the last lines of your code should be:def main ():
# your code inside of main
main()
- All of your own function definitions should be at the highest level of your program (i.e., no indenting). Though it is possible to do, functions should really not be DEFINED inside of other functions (they can be CALLED inside other functions though).
- A bit of arithmetic will help with the following requirements:
- You must use for loops.
- You must use a pen size between 20 and 30.
- The sides of your large outer squares must be 500 or larger.
- Your boustrophedon patterns must be non-overlapping and just touching.
- To design the first algorithm, try the design on a piece of paper, moving and turning your pen or cellphone as if it was a turtle. As you are considering the orientation and position of the turtle, you are considering changes of the turtle's state in the lingo of computer science. Note that you may design for algorithmic convenience, you need not have the exact number of turns shown in the videos.
- Write out the first design for your program in your Word document. Do the same for the second design.
- Decide which of these two designs you will implement. Explain the key reasons for your choice in your Word document.
- Using the typical program header for the class, create yourusernames-T3.py from your chosen algorithm.
- Once you have your implementation working, pretty it up by adding color, comments in the code, and text on the canvas.
- In your Word document, describe the challenges of this programming assignment. Did you struggle with getting the screen coordinates to work? Did the programming go smoothly after you selected the algorithms? Explain.
- Upload the program and the report to Moodle. The navigator should upload the Word document and the driver should submit the programs. If there is a third partner, they should upload the names of their other partners.